Debug Jest Unit Tests with VS Code breakpoints in React Native
An excellent way to debug your Jest unit tests is by adding breakpoints in the .test.ts
file and also in the .ts
one.
Visual Studio Code can support this by adding a new item in the configurations
list from the launch.json
file. This config will also read your jest.config.js
file.
Make sure you add the following config:
{
// put this inside /.vscode dir
// Use IntelliSense to learn about possible attributes.
// Hover to view descriptions of existing attributes.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"type": "node",
"request": "launch",
"name": "Jest: current file",
//"env": { "NODE_ENV": "test" },
"program": "${workspaceFolder}/node_modules/.bin/jest",
"args": ["${fileBasenameNoExtension}", "--config", "jest.config.js"],
"console": "integratedTerminal",
"disableOptimisticBPs": true,
"windows": {
"program": "${workspaceFolder}/node_modules/jest/bin/jest"
}
},
]
}
This config will run all the unit tests from the currently opened .test.ts
file. Add your breakpoints and turn on the debugger.
Here is a short demo:
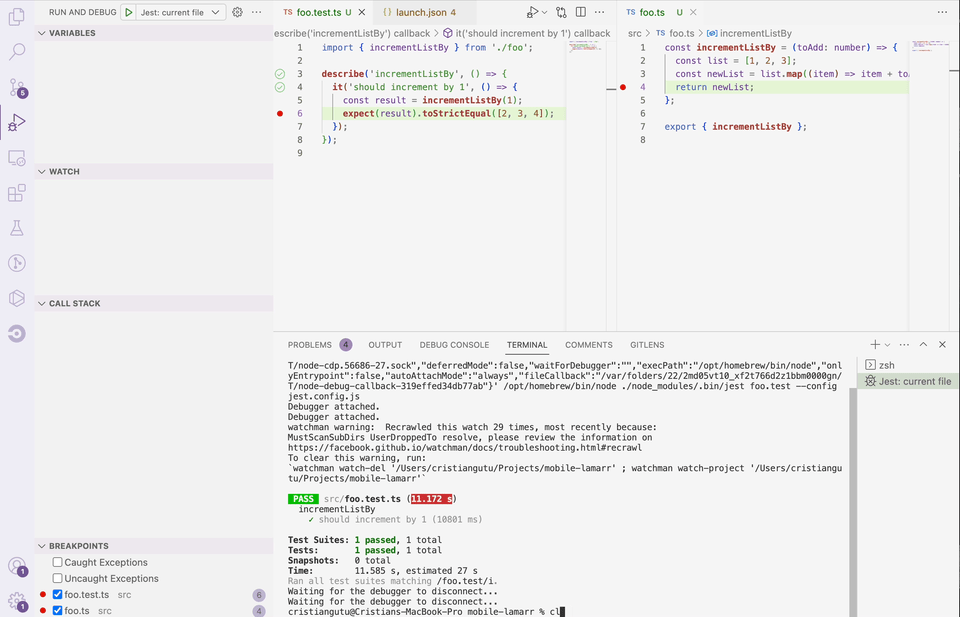
Another handy way to trigger those breakpoints is to have the vscode-jest
plugin installed.
https://github.com/jest-community/vscode-jest
This plugin has a lot of features:
- Starts Jest automatically for most projects with runnable jest configurations.
- Fully integrated with the VS Code TestExplorer.
- Supports both automatic and manual test runs at any level, and easy-switch via UI.
- Supports additional IntelliSense for jest methods.
- Show fails inline of the expect function, as well as in the problem inspector.
- View and update snapshots interactively.
- Help debug jest tests in VS Code.
- Show coverage information in files being tested.
- Supports monorepo, react, react-native, vue and various configurations/platforms.
With this plugin, you can also debug individual tests, take a look here:
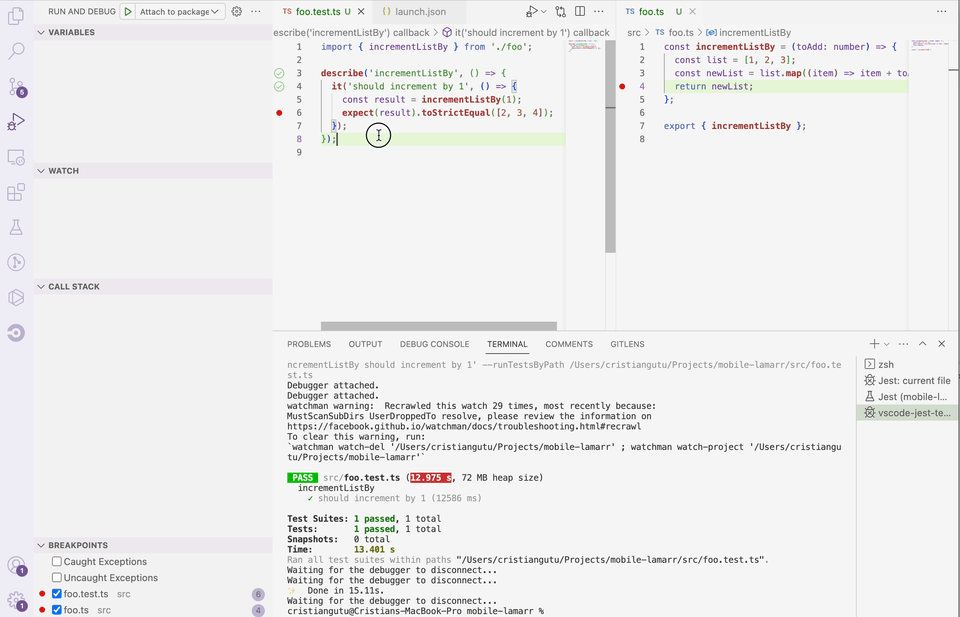
I hope this will help you when trying to figure out why some tests are not passing or why some mocks are not properly configured.